Back to Courses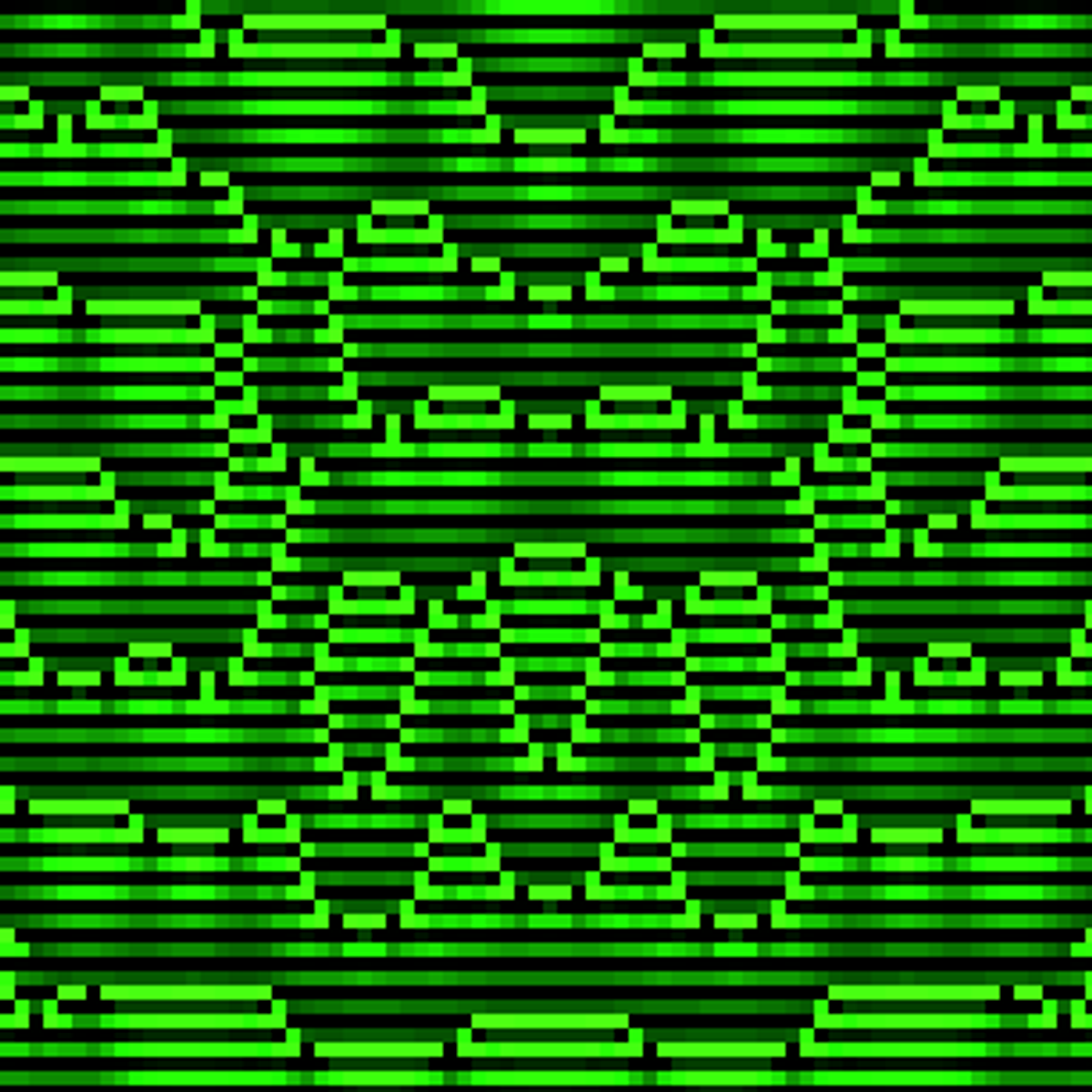
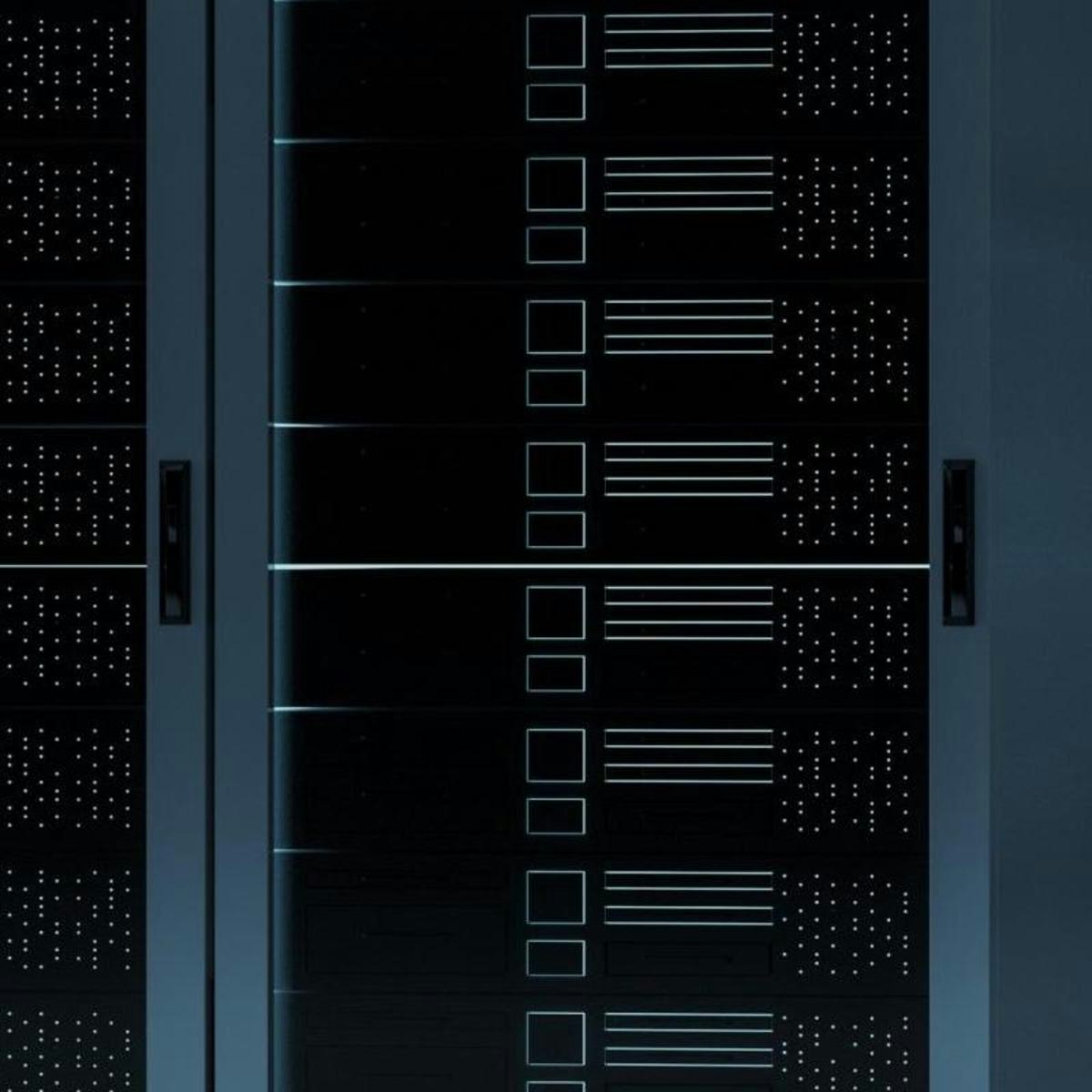
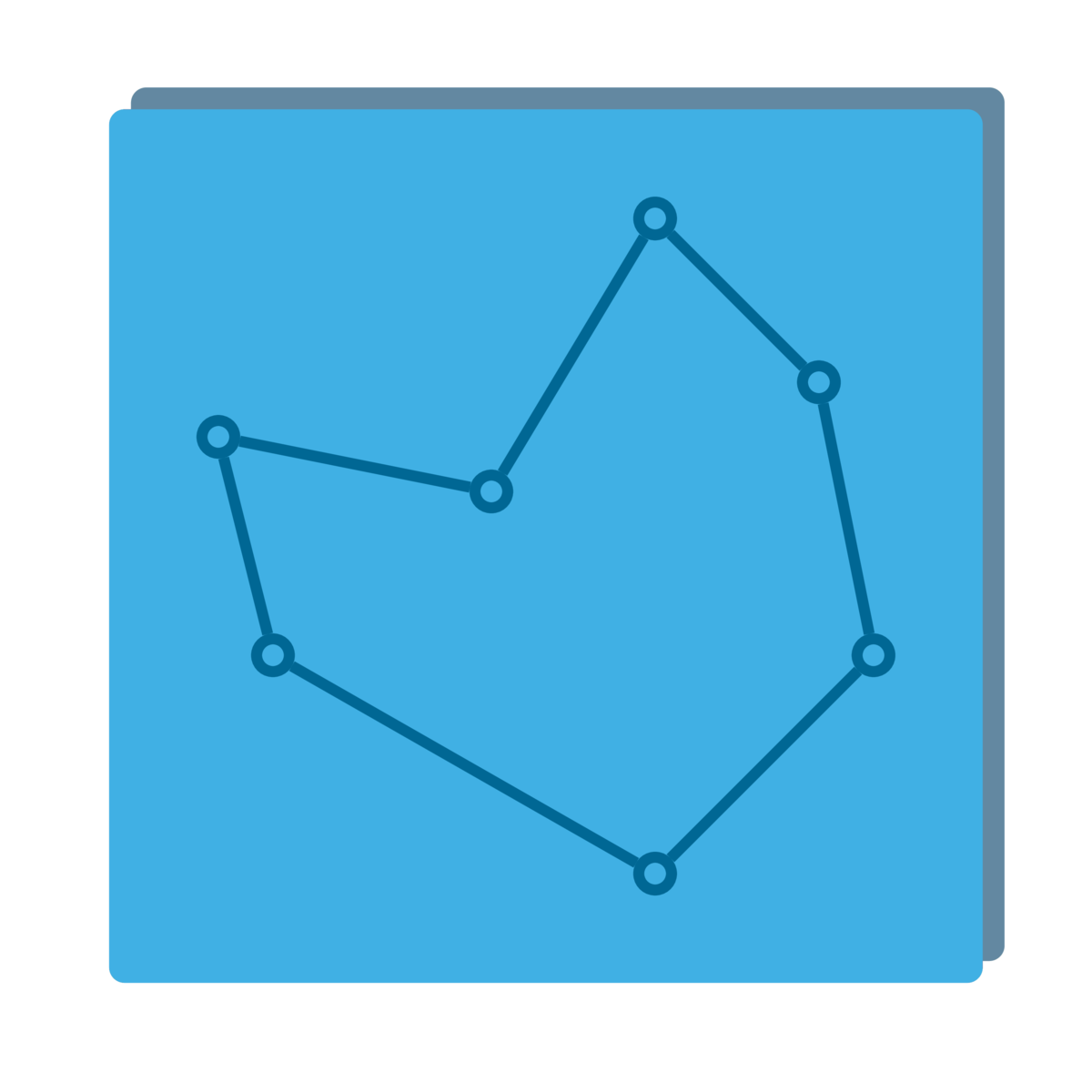
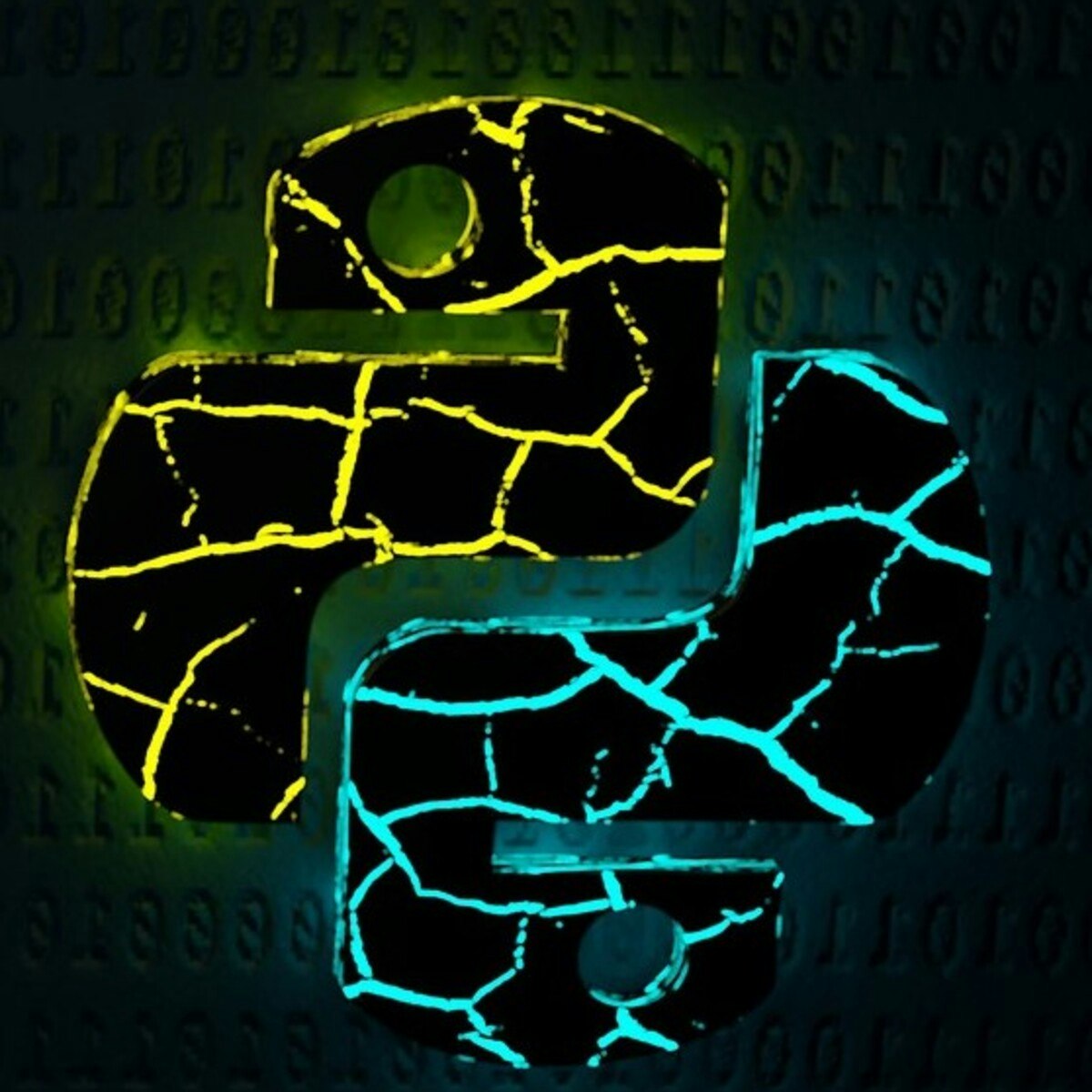
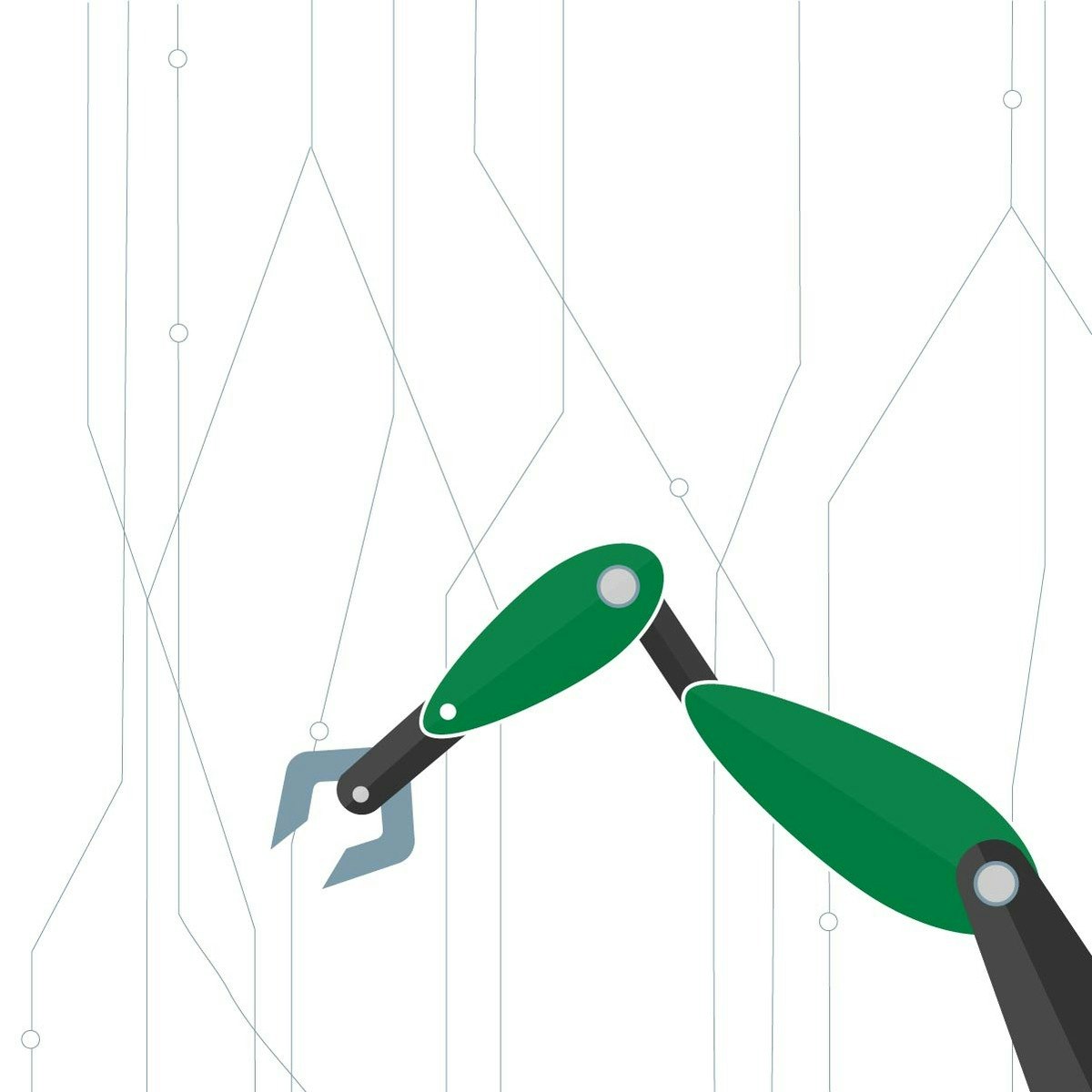
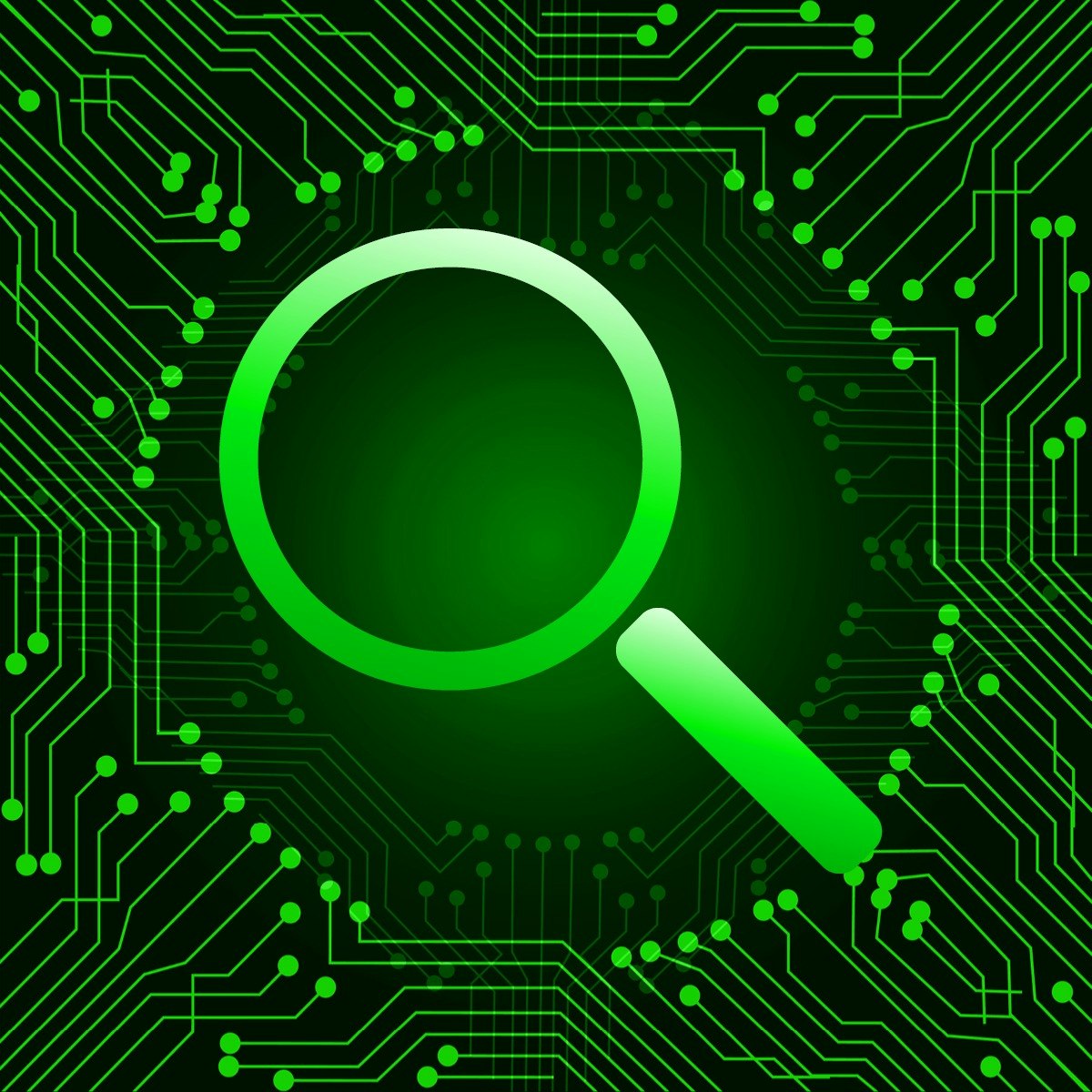
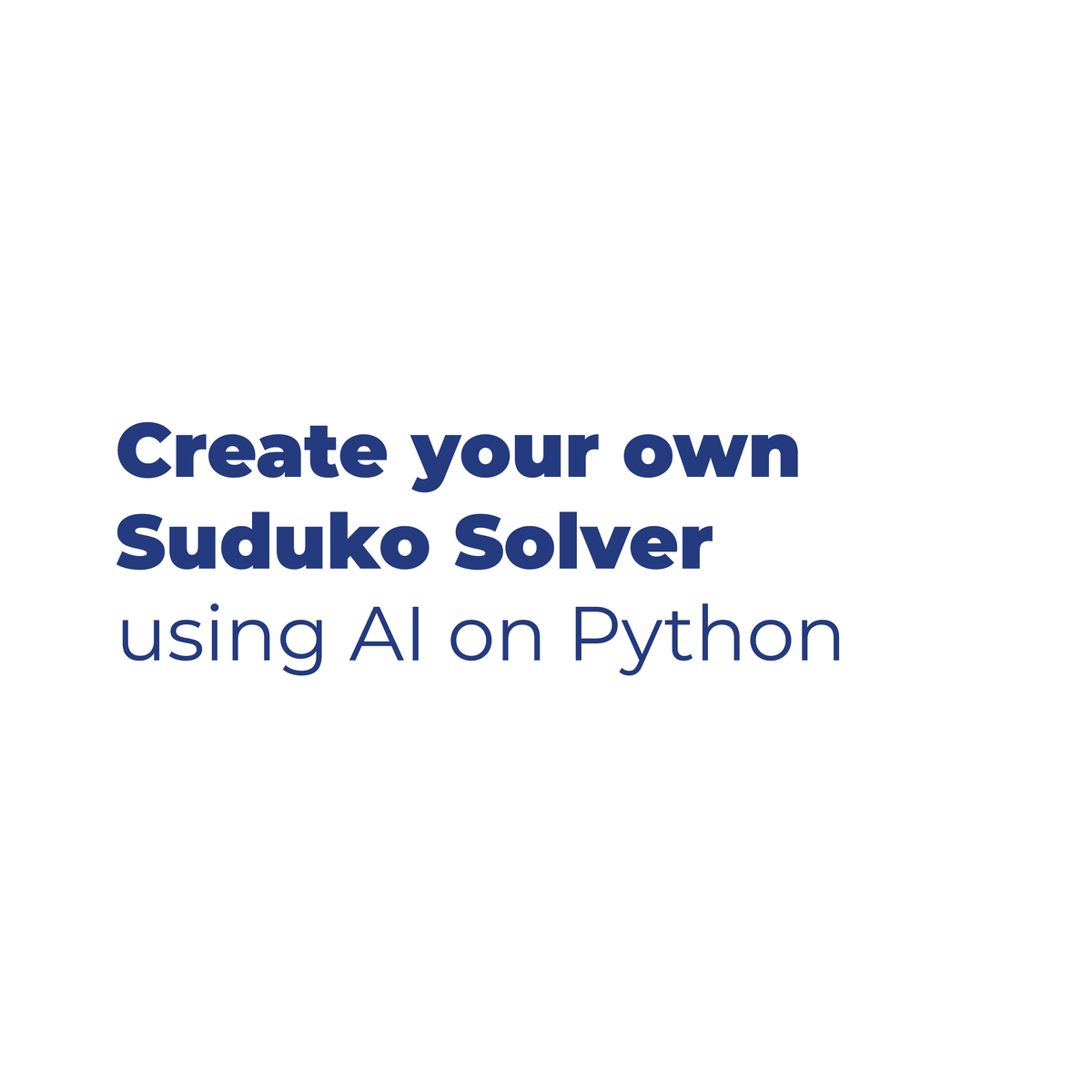
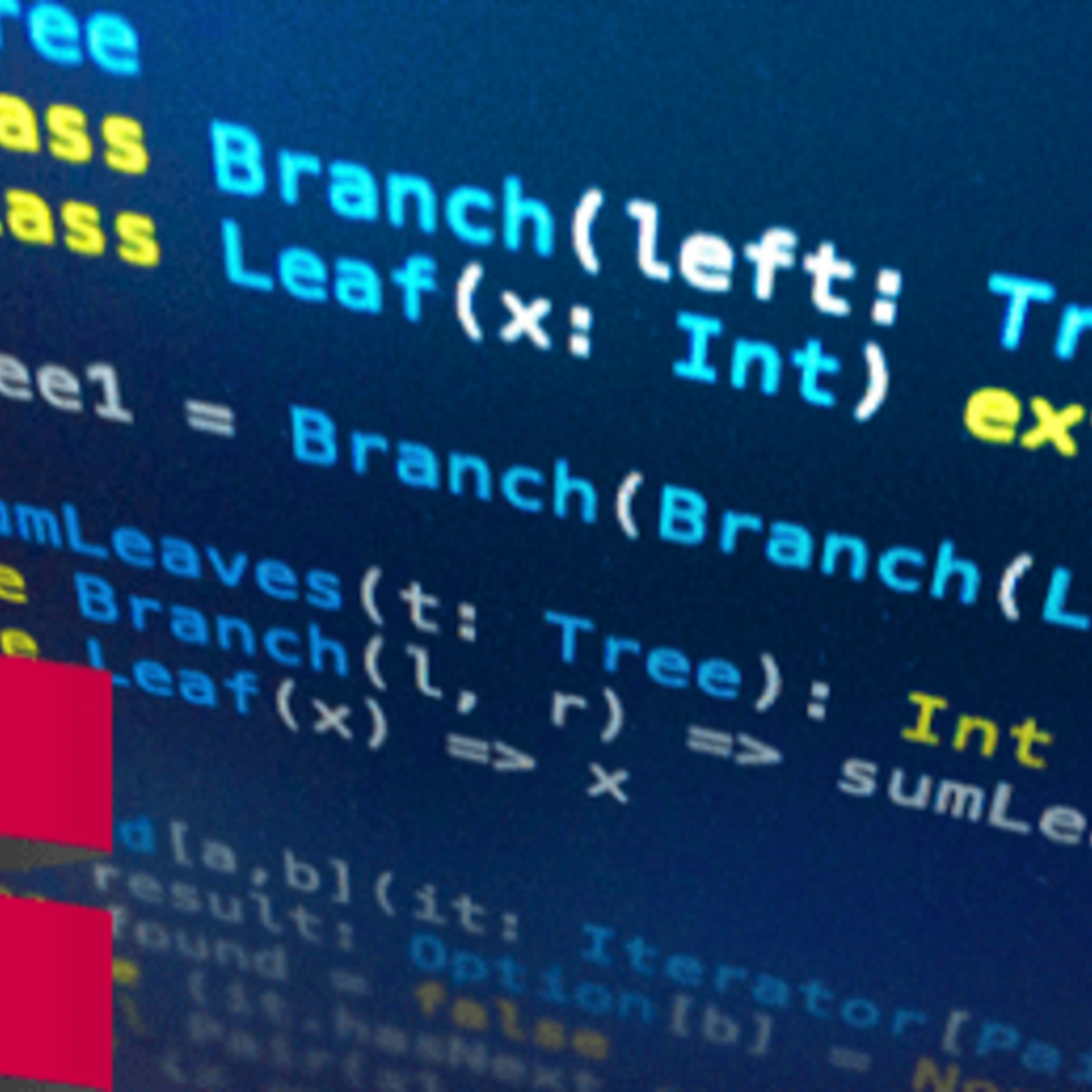
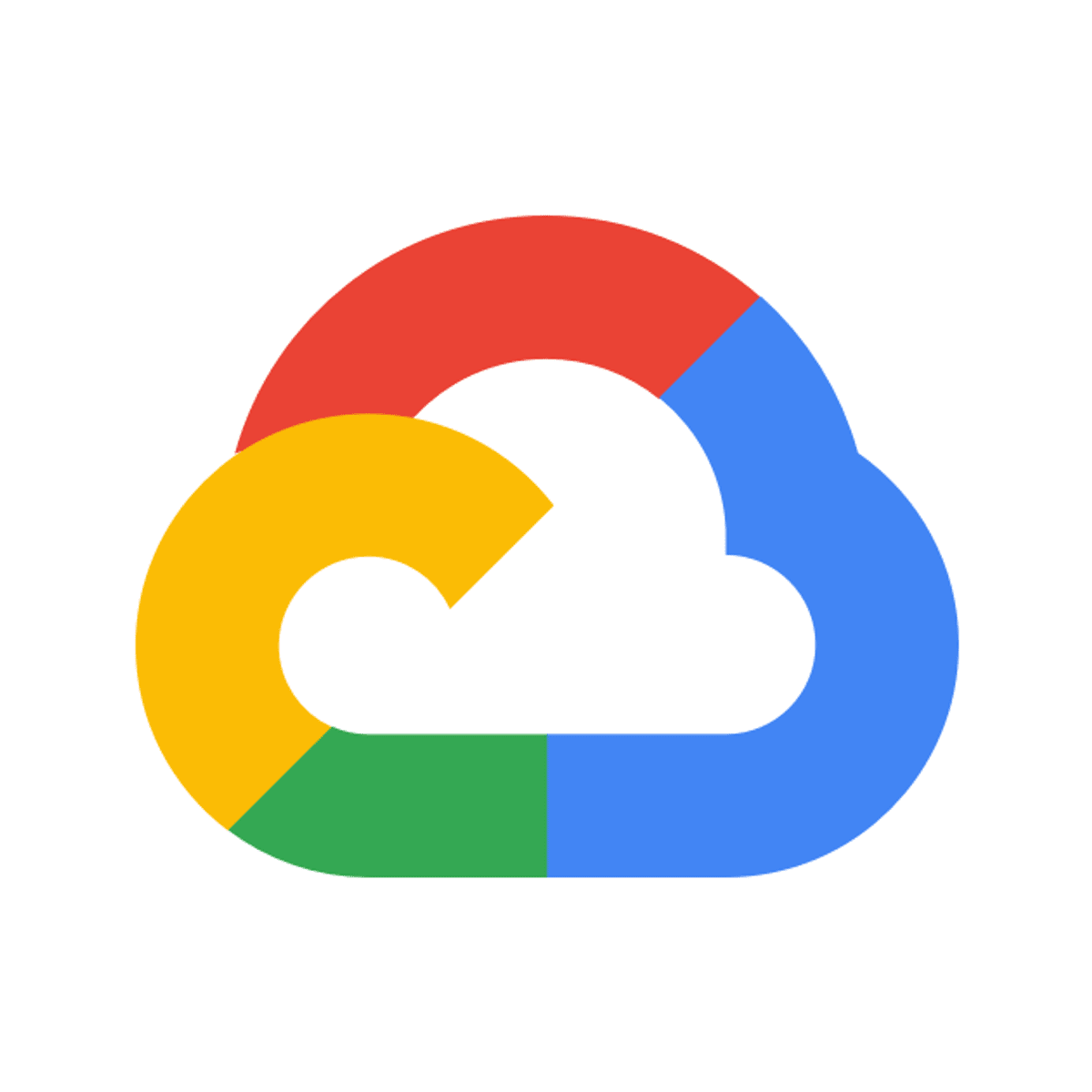
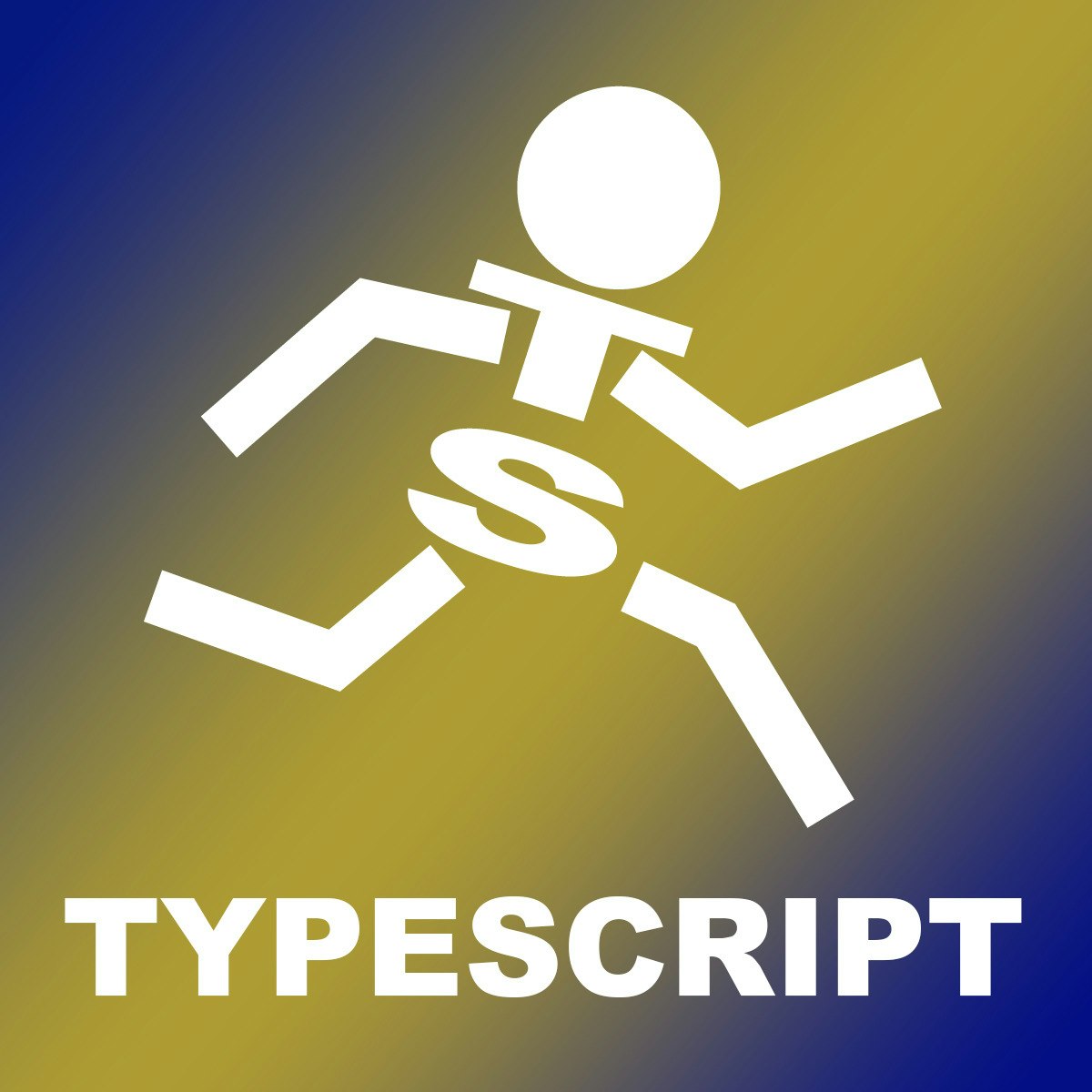
Algorithms Courses - Page 5
Showing results 41-50 of 326
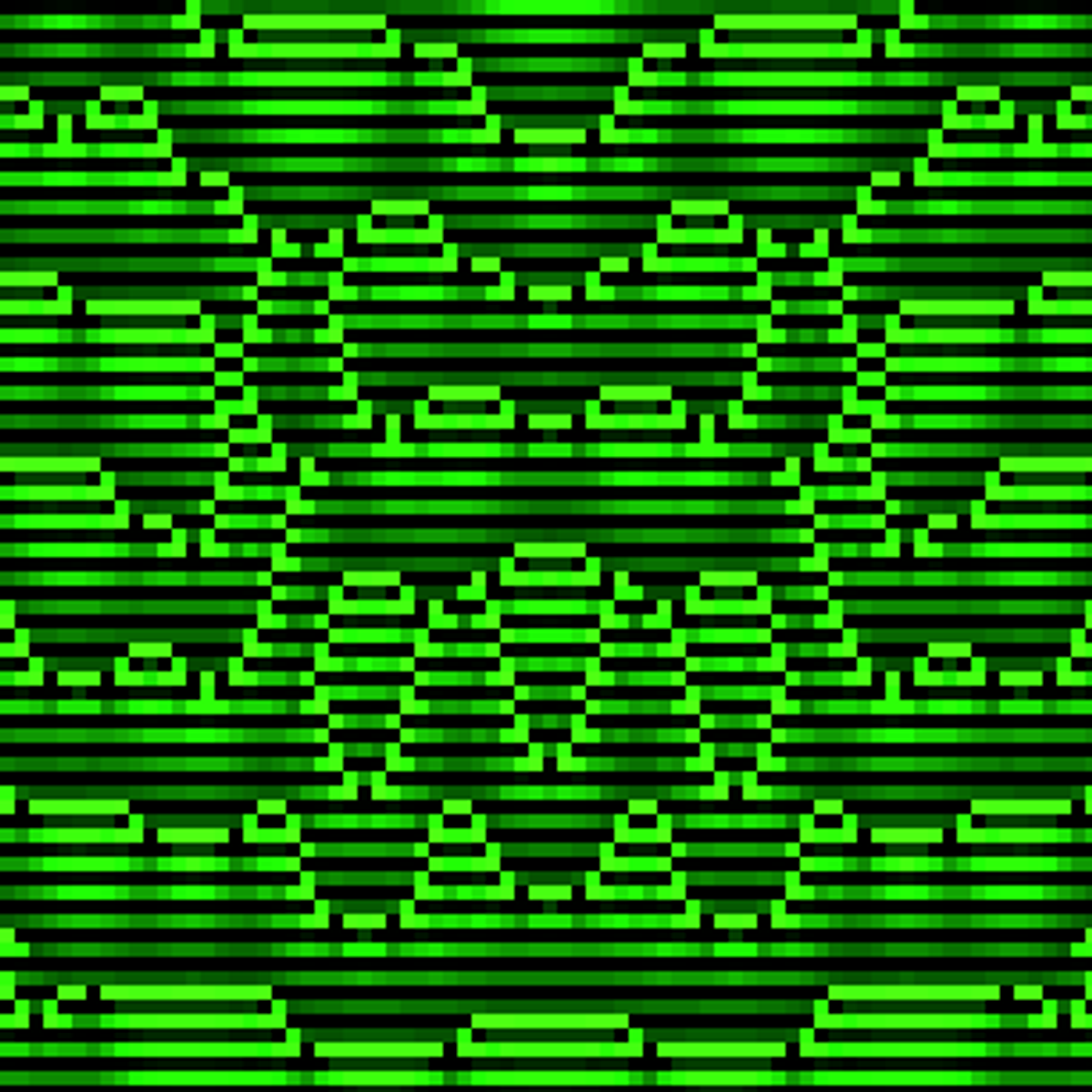
Introducing Cellular Automata
In this hands-on guided project you will be introduced to the wonders of Cellular Automata, a powerful modeling framework for the exploration of inter-scale dynamics. That is, how do simple local level rules give rise to global pattern formation and self-organization?
You will explore questions like this with the help of Golly and NetLogo, two rich simulations softwares for the creation of CA and agent-based models. Moreover, you will be exposed to cutting edge applications of Cellular Automata in the fields of Biology (Morphogenesis) and Physics.
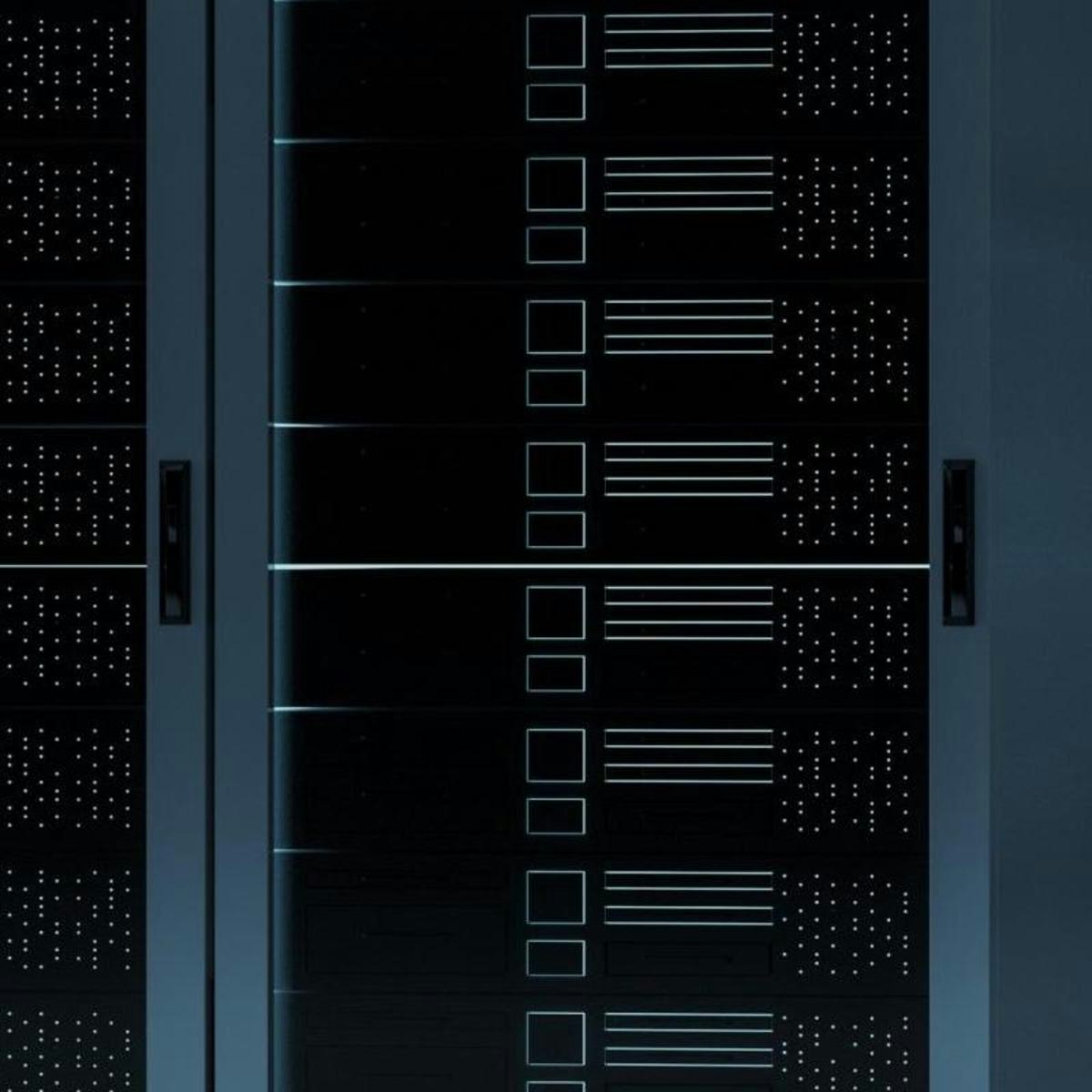
Introduction to High-Performance and Parallel Computing
This course introduces the fundamentals of high-performance and parallel computing. It is targeted to scientists, engineers, scholars, really everyone seeking to develop the software skills necessary for work in parallel software environments. These skills include big-data analysis, machine learning, parallel programming, and optimization. We will cover the basics of Linux environments and bash scripting all the way to high throughput computing and parallelizing code. We recommend you are familiar with either Fortran 90, C++, or Python to complete some of the programming assignments.
After completing this course, you will familiar with:
*The components of a high-performance distributed computing system
*Types of parallel programming models and the situations in which they might be used
*High-throughput computing
*Shared memory parallelism
*Distributed memory parallelism
*Navigating a typical Linux-based HPC environment
*Assessing and analyzing application scalability including weak and strong scaling
*Quantifying the processing, data, and cost requirements for a computational project or workflow
This course can be taken for academic credit as part of CU Boulder’s Master of Science in Data Science (MS-DS) degree offered on the Coursera platform. The MS-DS is an interdisciplinary degree that brings together faculty from CU Boulder’s departments of Applied Mathematics, Computer Science, Information Science, and others. With performance-based admissions and no application process, the MS-DS is ideal for individuals with a broad range of undergraduate education and/or professional experience in computer science, information science, mathematics, and statistics. Learn more about the MS-DS program at https://www.coursera.org/degrees/master-of-science-data-science-boulder.
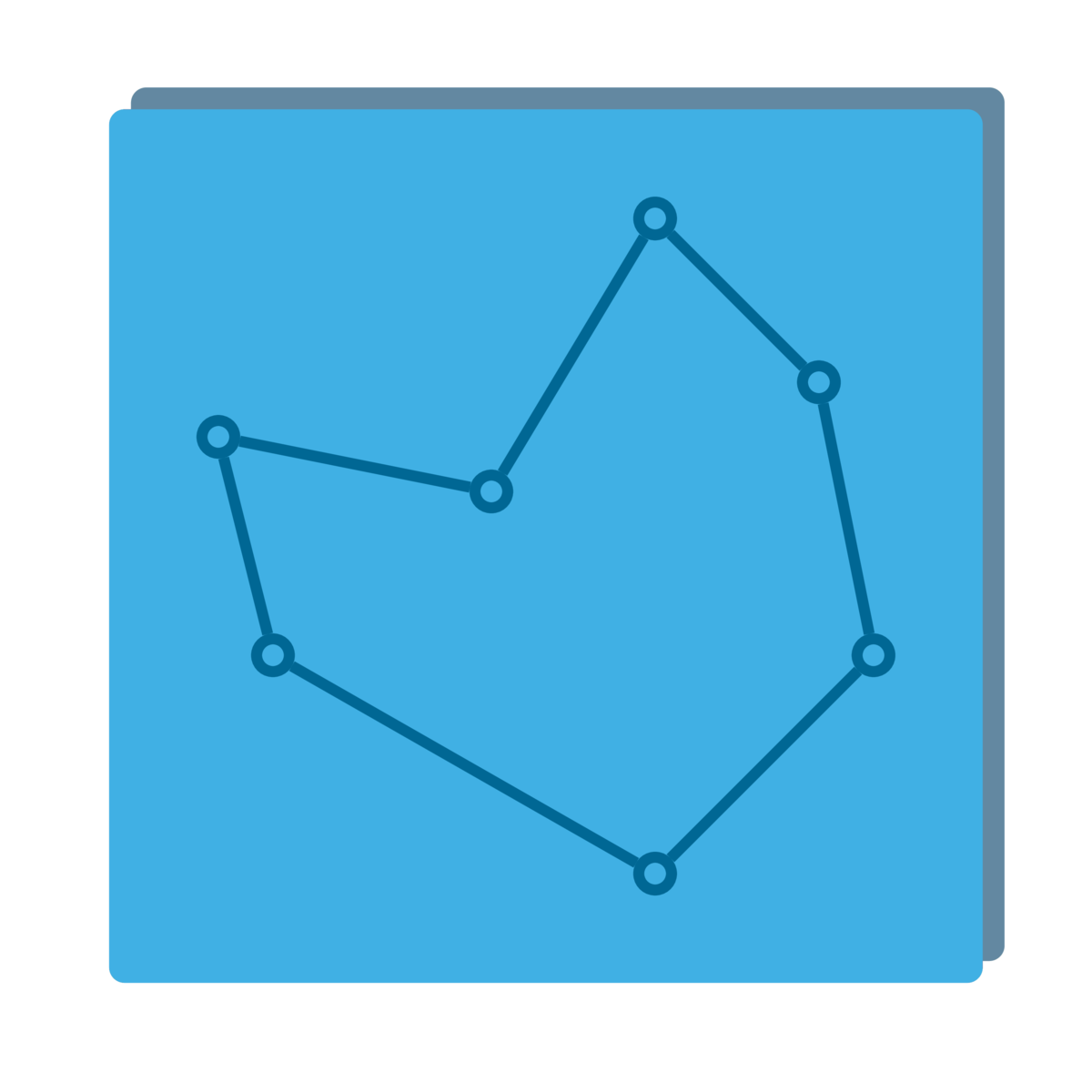
Delivery Problem
In this online course we’ll implement (in Python) together efficient programs for a problem needed by delivery companies all over the world millions times per day — the travelling salesman problem. The goal in this problem is to visit all the given places as quickly as possible. How to find an optimal solution to this problem quickly? We still don’t have provably efficient algorithms for this difficult computational problem and this is the essence of the P versus NP problem, the most important open question in Computer Science. Still, we’ll implement several solutions for real world instances of the travelling salesman problem.
While designing these solutions, we will rely heavily on the material learned in the courses of the specialization: proof techniques, combinatorics, probability, graph theory. We’ll see several examples of using discrete mathematics ideas to get more and more efficient solutions.
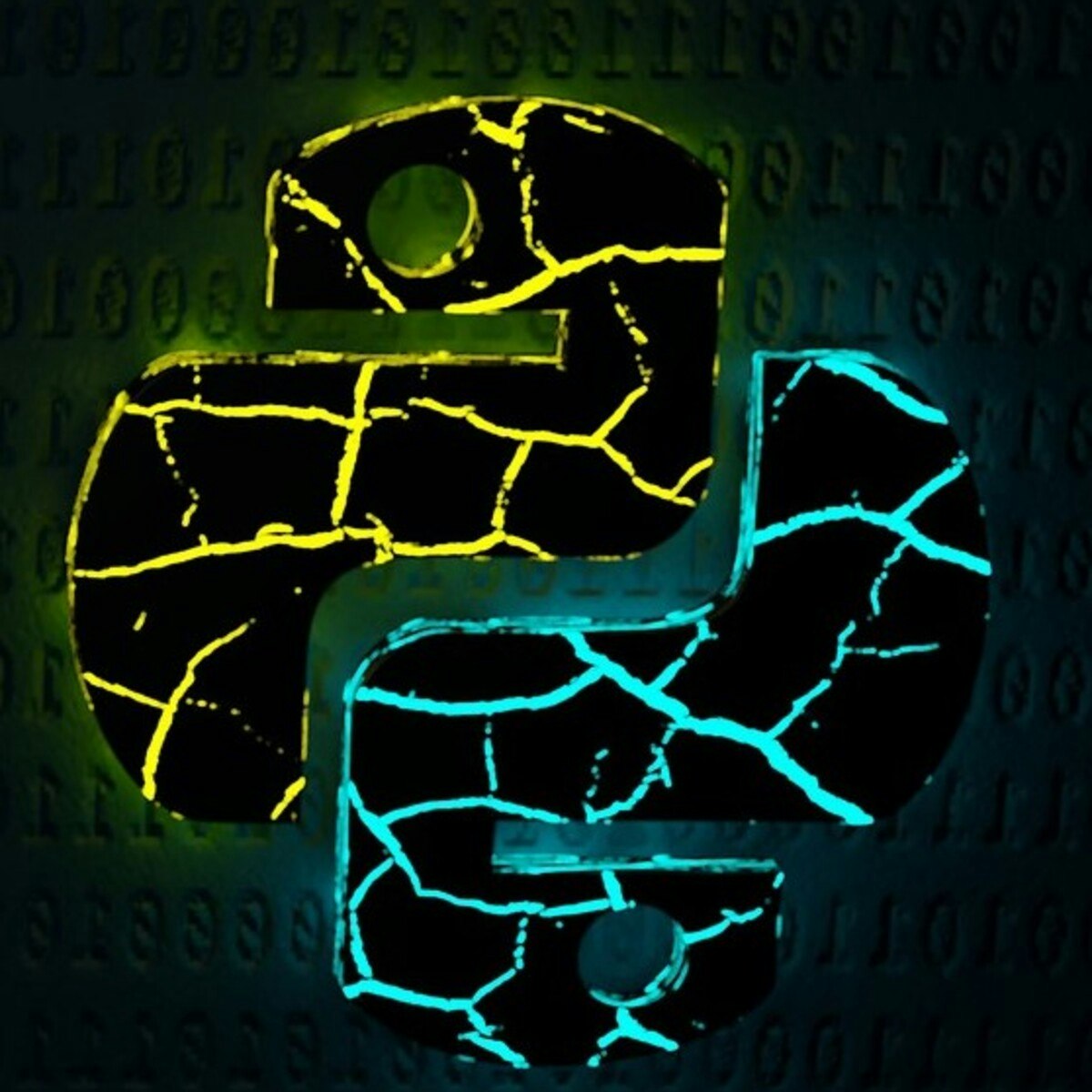
Big-O Time Complexity in Python Code
In the field of data science, the volumes of data can be enormous, hence the term Big Data. It is essential that algorithms operating on these data sets operate as efficiently as possible. One measure used is called Big-O time complexity. It is often expressed not in terms of clock time, but rather in terms of the size of the data it is operating on. For example, in terms of an array of size N, an algorithm may take N^2 operations to complete. Knowing how to calculate Big-O gives the developer another tool to make software as good as it can be and provides a means to communicate performance when reviewing code with others.
In this course, you will analyze several algorithms to determine Big-O performance. You will learn how to visualize the performance using the graphing module pyplot.
Note: This course works best for learners who are based in the North America region. We’re currently working on providing the same experience in other regions.
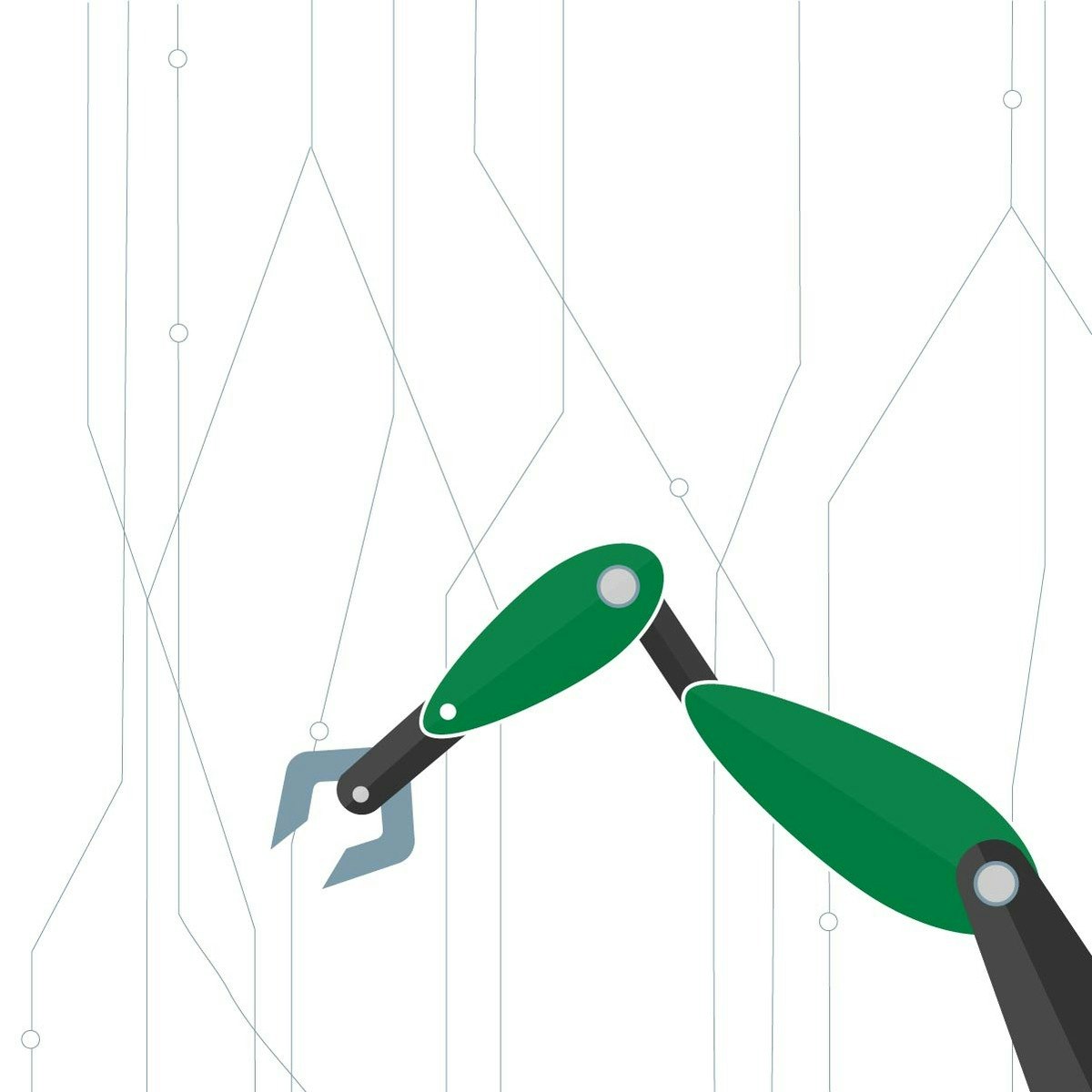
Prediction and Control with Function Approximation
In this course, you will learn how to solve problems with large, high-dimensional, and potentially infinite state spaces. You will see that estimating value functions can be cast as a supervised learning problem---function approximation---allowing you to build agents that carefully balance generalization and discrimination in order to maximize reward. We will begin this journey by investigating how our policy evaluation or prediction methods like Monte Carlo and TD can be extended to the function approximation setting. You will learn about feature construction techniques for RL, and representation learning via neural networks and backprop. We conclude this course with a deep-dive into policy gradient methods; a way to learn policies directly without learning a value function. In this course you will solve two continuous-state control tasks and investigate the benefits of policy gradient methods in a continuous-action environment.
Prerequisites: This course strongly builds on the fundamentals of Courses 1 and 2, and learners should have completed these before starting this course. Learners should also be comfortable with probabilities & expectations, basic linear algebra, basic calculus, Python 3.0 (at least 1 year), and implementing algorithms from pseudocode.
By the end of this course, you will be able to:
-Understand how to use supervised learning approaches to approximate value functions
-Understand objectives for prediction (value estimation) under function approximation
-Implement TD with function approximation (state aggregation), on an environment with an infinite state space (continuous state space)
-Understand fixed basis and neural network approaches to feature construction
-Implement TD with neural network function approximation in a continuous state environment
-Understand new difficulties in exploration when moving to function approximation
-Contrast discounted problem formulations for control versus an average reward problem formulation
-Implement expected Sarsa and Q-learning with function approximation on a continuous state control task
-Understand objectives for directly estimating policies (policy gradient objectives)
-Implement a policy gradient method (called Actor-Critic) on a discrete state environment
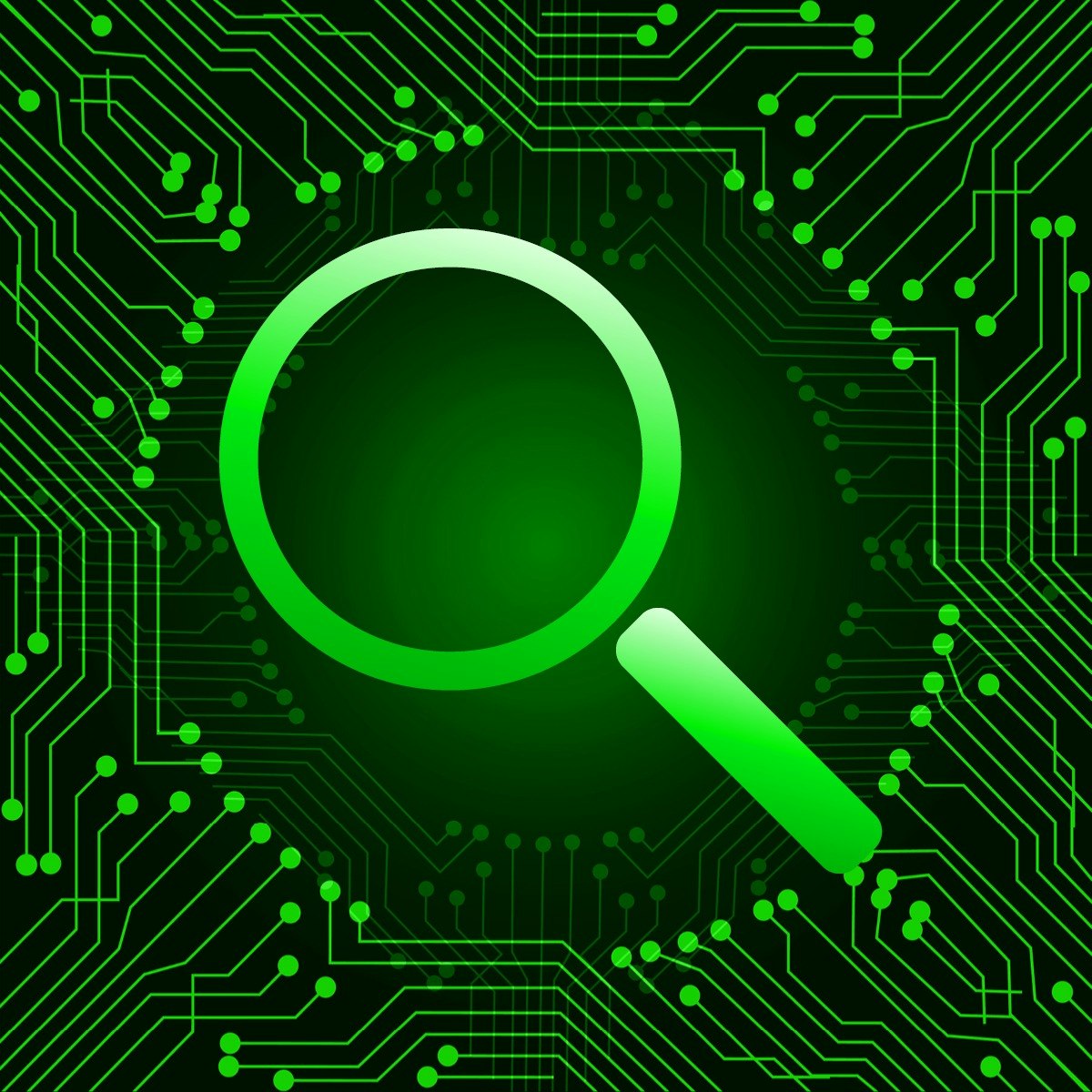
Introduction to Applied Cryptography
This course is a non-mathematical introduction to the role that cryptography plays in providing digital security for everyday applications such as the internet, mobile phones, wireless networks and cryptocurrency.
In this introductory course you will develop an understanding of the functionality and purpose of the main cryptographic tools we use today. You will learn how to make decisions about which cryptographic tools are most appropriate to deploy in specific settings. You will also explore the wider infrastructure surrounding cryptography and how this impacts the overall security of systems deploying cryptography.
Cryptography provides the core toolkit that underpins most digital security technologies. An understanding of what cryptography does, and its limitations, is critical to developing a wider appreciation of the security of everyday digital applications. Since cryptography provides tools for atomic security services such as confidentiality and data integrity, an appreciation of cryptography will also equips you with a fundamental understanding of what security means in cyberspace.
Learning outcomes for the module.
By the end of this module learners will be able to:
1. Explain the precise role that cryptography plays in the security of any digital system.
2. Appreciate the breadth of use of cryptography to support security of digital systems.
3. Identify core concepts and terminology concerning use of cryptography
4. Assess the points of vulnerability relating to cryptography in any digital system deploying it.
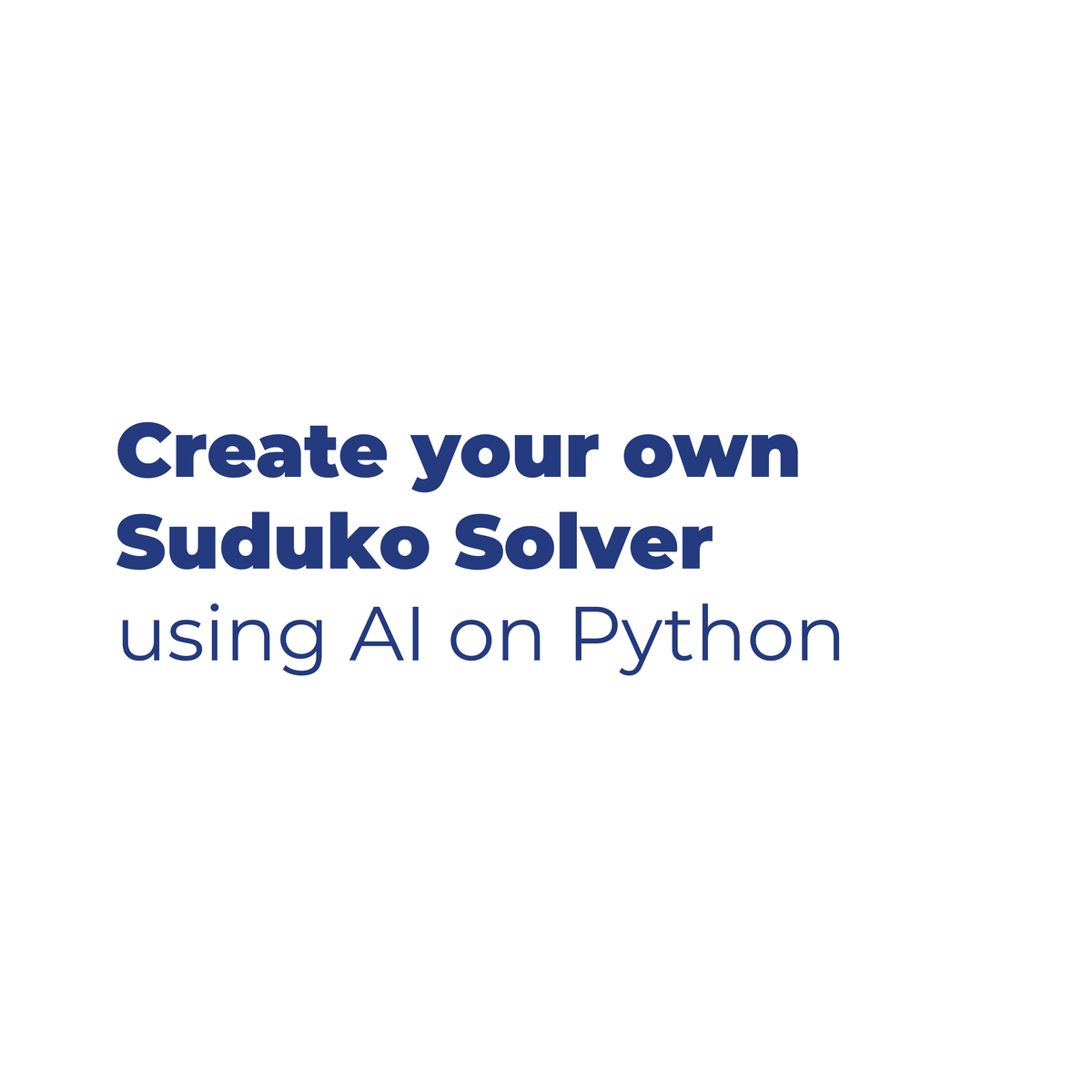
Create your own Sudoku Solver using AI and Python
In this 1-hour long project-based course, you will create a Sudoku game solver using Python. This problem is an example of what is called a Constraint Satisfaction Problem (CSP) in the field of Artificial Intelligence. CSP is a mathematical problem that must satisfy a number of constraints or limitations all the time.
In this project, You will use the Backtracking algorithm to solve CSPs, in a Sudoku game. Backtracking is a recursive algorithm that tries to build a solution incrementally, removing solutions that fail to satisfy the constraints. Eventually, you will be able to use the knowledge acquired from this project on far more complex projects that employ these technologies.
Note: This course works best for learners who are based in the North America region. We’re currently working on providing the same experience in other regions.
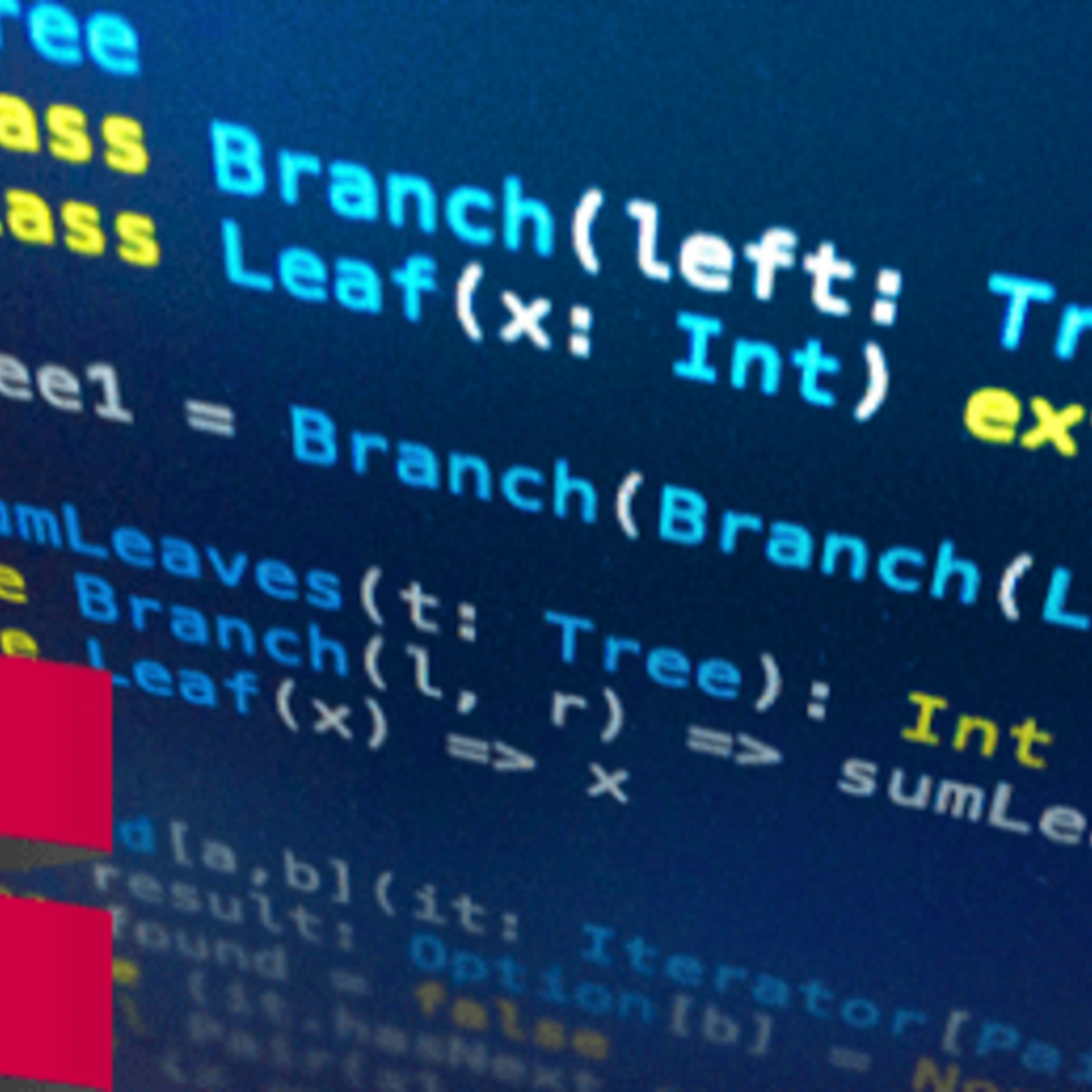
Big Data Analysis with Scala and Spark
Manipulating big data distributed over a cluster using functional concepts is rampant in industry, and is arguably one of the first widespread industrial uses of functional ideas. This is evidenced by the popularity of MapReduce and Hadoop, and most recently Apache Spark, a fast, in-memory distributed collections framework written in Scala. In this course, we'll see how the data parallel paradigm can be extended to the distributed case, using Spark throughout. We'll cover Spark's programming model in detail, being careful to understand how and when it differs from familiar programming models, like shared-memory parallel collections or sequential Scala collections. Through hands-on examples in Spark and Scala, we'll learn when important issues related to distribution like latency and network communication should be considered and how they can be addressed effectively for improved performance.
Learning Outcomes. By the end of this course you will be able to:
- read data from persistent storage and load it into Apache Spark,
- manipulate data with Spark and Scala,
- express algorithms for data analysis in a functional style,
- recognize how to avoid shuffles and recomputation in Spark,
Recommended background: You should have at least one year programming experience. Proficiency with Java or C# is ideal, but experience with other languages such as C/C++, Python, Javascript or Ruby is also sufficient. You should have some familiarity using the command line. This course is intended to be taken after Parallel Programming: https://www.coursera.org/learn/parprog1.
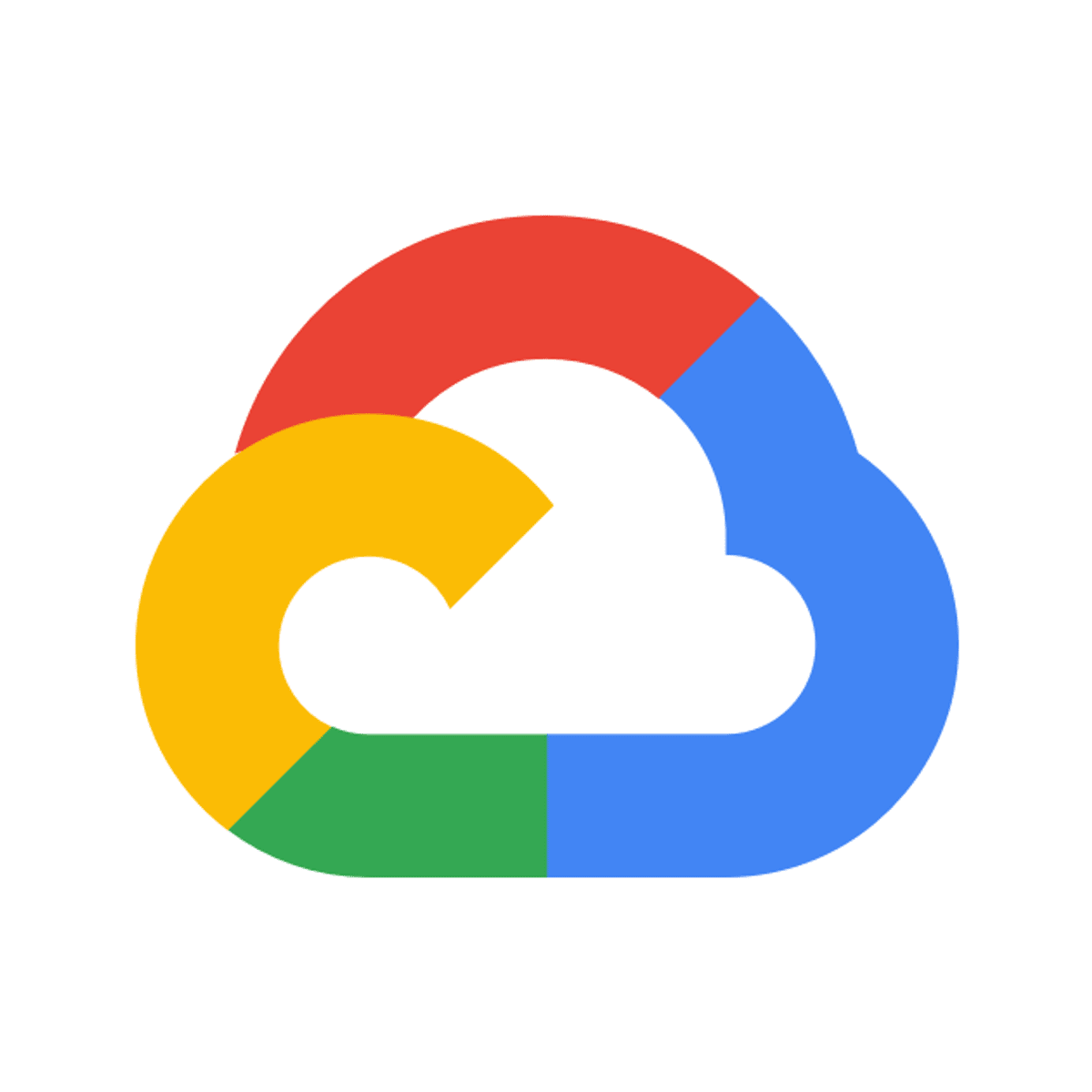
APIs Explorer: Create and Update a Cluster
This is a self-paced lab that takes place in the Google Cloud console.
In this lab you’ll learn how to use an inline Google APIs Explorer template to call the Cloud Dataproc API to create a cluster, run a simple Spark job in the cluster, and update the cluster.
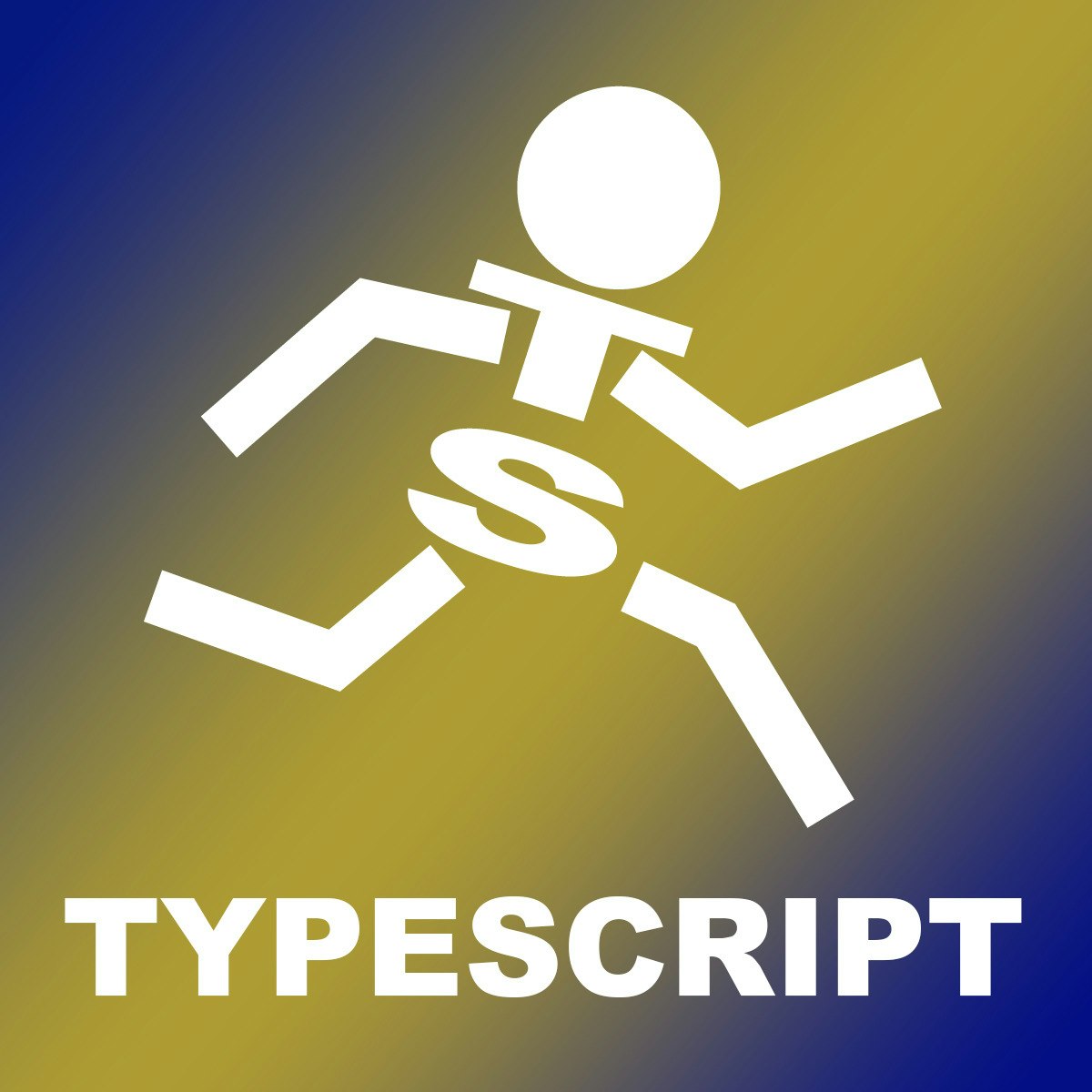
Get Up and Running with TypeScript
In this 1.5 hour class you will learn about TypeScript and start writing code that compile to JavaScript. You will also debug a small JavaScript webpage using TypeScript. At the end of this class you will have everything you need to start using TypeScript!
Required: Basic to Intermediate JavaScript
Popular Internships and Jobs by Categories
Browse
© 2024 BoostGrad | All rights reserved